Primary Constructors in C# 12
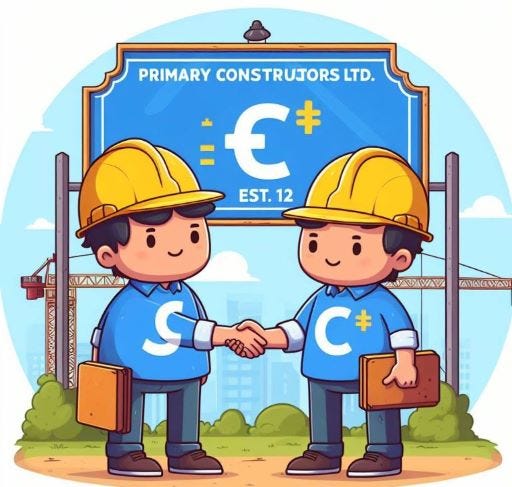
Today, we’re going to explore a new feature introduced in C# 12, part of the .NET 8 release, that has simplified object initialization and made our code more concise and readable: Primary Constructors.
What are Primary Constructors?
Primary Constructors are a new feature in C# 12 that allows you to define and initialize properties directly within the constructor parameter list. This feature eliminates the need for repeated code and makes your code more clean, concise and readable.
Understanding Primary Constructors
You can add parameters to a class or struct declaration to create a primary constructor. Primary constructor parameters are in scope throughout the class definition. It’s important to view primary constructor parameters as parameters even though they are in scope throughout the class definition.
Here are some rules that clarify they’re parameters:
- Primary constructor parameters may not be stored if they aren’t needed.
- Primary constructor parameters aren’t members of the class.
- Primary constructor parameters can be assigned to.
- Primary constructor parameters don’t become properties, except in record types.
Before Primary Constructors
Before the introduction of primary constructors in C# 12, we had to explicitly define constructors and map incoming parameters to private fields or properties within the class or struct. Here’s an example of how we used to create a class with a constructor:
public class Employee
{
public string FirstName { get; set; }
public string LastName { get; set; }
public DateTime HireDate { get; set; }
public decimal Salary { get; set; }
public Employee(string firstName, string lastName, DateTime hireDate, decimal salary)
{
FirstName = firstName;
LastName = lastName;
HireDate = hireDate;
Salary = salary;
}
}
Using Primary Constructors
With the introduction of primary constructors in C# 12, we can now define and initialize properties directly within the constructor parameter list, eliminating the need for repeated code and making our code more concise and readable. Here’s how we can refactor the above Employee
class using primary constructors:
public class Employee(string firstName, string lastName, DateTime hireDate, decimal salary)
{
public string FirstName { get; set; } = firstName;
public string LastName { get; set; } = lastName;
public DateTime HireDate { get; set; } = hireDate;
public decimal Salary { get; set; } = salary;
}
In this refactored Employee class, we’ve added parameters to the class declaration to create a primary constructor. These parameters are used to initialize the properties directly. This new syntax has made the code more concise and eliminated the redundancy of declaring parameters in a separate constructor and then assigning them to properties.
Conclusion
Primary constructors in C# 12 have simplified object initialization by allowing us to define and initialize properties directly within the constructor parameter list. This feature has made our code more concise and readable, and has eliminated the redundancy of declaring parameters in a separate constructor and then assigning them to properties..
C# 12 is part of the .NET 8 release, which comes with many new features and improvements, making it a powerful platform for building a variety of applications. We will keep exploring these features. Happy coding!